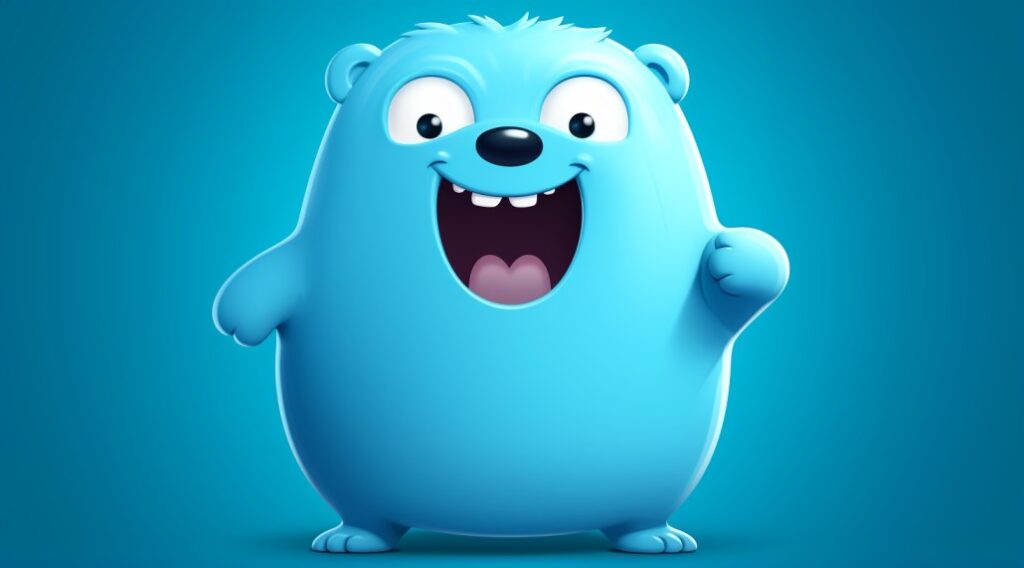
Converting Go Structs to Maps: A Practical Guide
Table of Contents
- The Magic of JSON Encoding and Decoding
- Converting a Struct to a Map
- Converting a Map to a Struct
- Conclusion
Do you find yourself needing to switch between Go structs and maps? In this practical guide, we’ll explore a straightforward method for converting Go structs to maps and vice versa using JSON encoding and decoding. Let’s dive right in!
The Magic of JSON Encoding and Decoding
JSON (JavaScript Object Notation) provides a simple yet powerful way to encode and decode data. In Go, we can leverage this magic to seamlessly transform our data structures.
Converting a Struct to a Map
Let’s start with the process of converting a Go struct to a map:
package main
import (
"encoding/json"
"fmt"
)
// 1. Create Your Struct
type MyStruct struct {
Field1 string
Field2 int
}
func main() {
myStruct := MyStruct{"Value1", 42}
// 2. Marshal the struct into a JSON string
jsonData, err := json.Marshal(myStruct)
if err != nil {
fmt.Println("Error marshaling struct:", err)
return
}
// 3. Unmarshal the JSON string into a map
var dataMap map[string]interface{}
err = json.Unmarshal(jsonData, &dataMap)
if err != nil {
fmt.Println("Error unmarshaling JSON:", err)
return
}
// Print the resulting map
fmt.Println(dataMap)
}
Output :
map[Field1:Value1 Field2:42]
1. Create Your Struct: Begin with a struct, like the one below.
2. Marshal to JSON: Use the json.Marshal() function to convert your struct to a JSON string.
3. Unmarshal JSON to Map: Unmarshal the JSON string into a map.
That’s it! You’ve successfully converted your struct into a map.
Converting a Map to a Struct
Converting a map back to a struct is just as easy:
package main
import (
"encoding/json"
"fmt"
)
type MyStruct struct {
Field1 string
Field2 int
}
func main() {
// 1. Create Your Map
dataMap := map[string]interface{}{
"Field1": "Value1",
"Field2": 42,
}
// 2. Marshal the map into a JSON string
jsonData, err := json.Marshal(dataMap)
if err != nil {
fmt.Println("Error marshaling map:", err)
return
}
// 3. Unmarshal the JSON string into a struct
var myStruct MyStruct
err = json.Unmarshal(jsonData, &myStruct)
if err != nil {
fmt.Println("Error unmarshaling JSON:", err)
return
}
// Print the resulting struct
fmt.Println(myStruct)
}
Output :
{Value1 42}
1. Create Your Map: Begin with a map containing the data you want to convert.
2. Marshal to JSON: Similar to the previous process, marshal your map to a JSON string.
3. Unmarshal JSON to Struct: Unmarshal the JSON string into your struct.
Conclusion
Converting Go structs to maps (and vice versa) doesn’t have to be a complex task. With JSON encoding and decoding, you can seamlessly switch between these data representations. So whether you’re working with APIs, databases, or any data source, you’re now armed with a practical and efficient solution.
Happy coding!