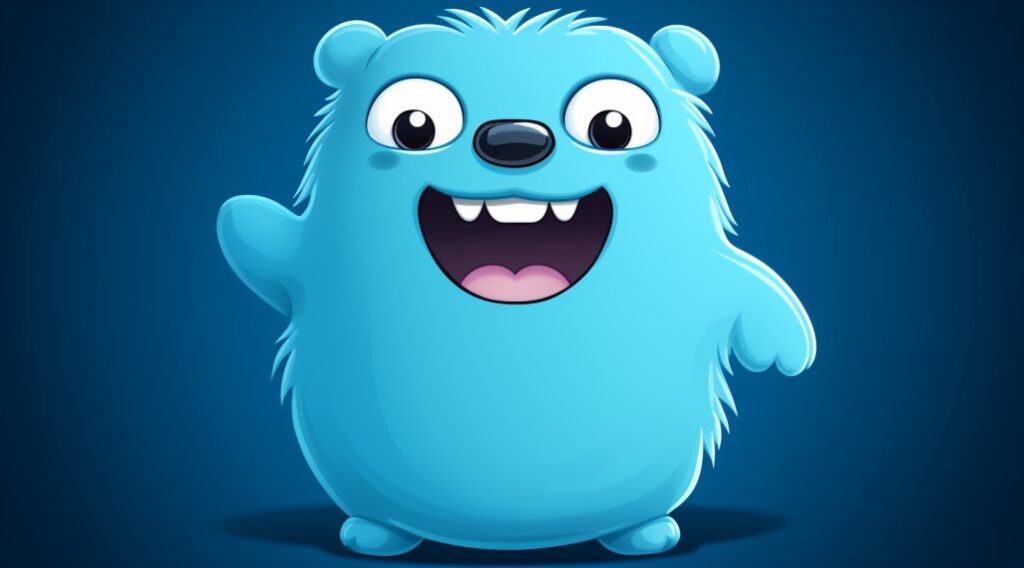
Base64 Encoding and Decoding in Golang: Examples
Table of Contents
- What is Base64 Encoding and Decoding?
- How to Base64 Encode and Decode in Golang
- Base64 Encoding in Golang
- Base64 Decoding in Golang
- Explanation
- The Result
- Conclusion
Base64 encoding and decoding in Golang are crucial skills for any developer. These techniques enable the representation of binary data in a human-readable format and its retrieval back to its original form. In this article, we’ll explore how to perform both Base64 encoding and decoding in Golang with easy-to-follow examples.
What is Base64 Encoding and Decoding?
Base64 encoding is the process of converting binary data into a plain text format, making it suitable for purposes like data transmission and storage. Decoding, on the other hand, reverses this process, converting Base64-encoded data back into its original binary form.
How to Base64 Encode and Decode in Golang
In Golang, we can use the encoding/base64 package to easily perform both encoding and decoding. Let’s take a look at the code snippets for each:
Base64 Encoding in Golang
package main
import (
"encoding/base64"
"fmt"
)
func main() {
data := []byte("Hello, World!")
// Encode data to Base64
encoded := base64.StdEncoding.EncodeToString(data)
fmt.Println(encoded)
}
Output :
SGVsbG8sIFdvcmxkIQ==
Base64 Decoding in Golang
package main
import (
"encoding/base64"
"fmt"
)
func main() {
encoded := "SGVsbG8sIFdvcmxkIQ=="
// Decode Base64-encoded data
decoded, err := base64.StdEncoding.DecodeString(encoded)
if err != nil {
fmt.Println("Error decoding:", err)
return
}
fmt.Println(string(decoded))
}
Output :
Hello, World!
Explanation
- For encoding, we use
base64.StdEncoding.EncodeToString()
to convert data to Base64. - For decoding,
base64.StdEncoding.DecodeString()
is employed to retrieve the original data.
The Result
After encoding, you’ll receive a Base64 encoded string. Upon decoding, you’ll retrieve the original data.
Conclusion
Mastering Base64 encoding and decoding in Golang opens up a world of possibilities for working with binary data. These techniques are essential for various applications, including web development and data storage. By utilizing the encoding/base64
package, Golang provides a straightforward solution for handling these operations efficiently. Remember, these skills are invaluable tools in any developer’s arsenal.