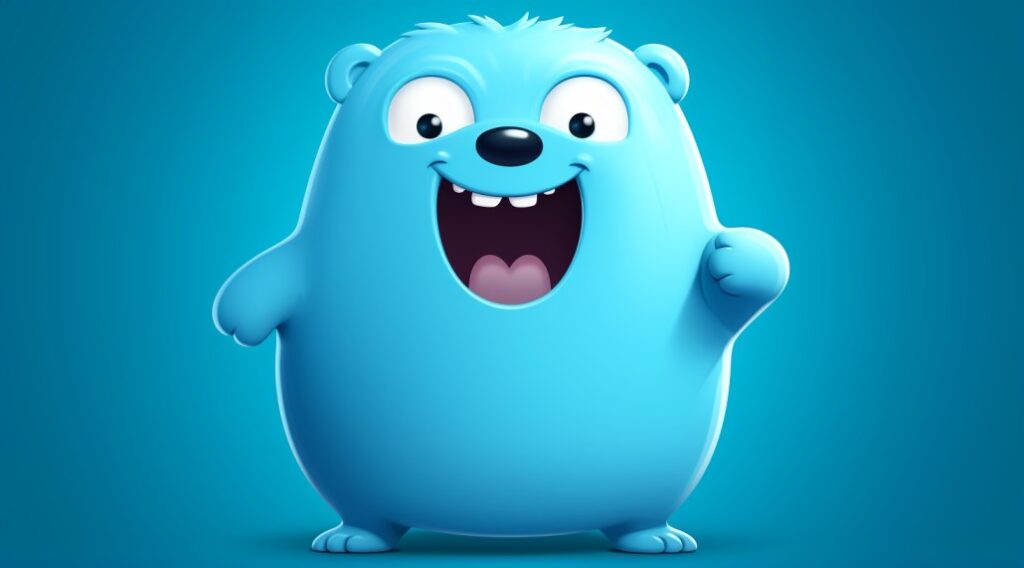
Working with Hashmaps in Golang
Introduction
In Go (often referred to as Golang) there is no built-in “hashmaps” data structure per se, but you can implement a hashmap-like data structure using the built-in map type. A map in Go is a built-in data structure that provides an efficient way to store key-value pairs. In this article, we will explore how to declare, initialize, insert, retrieve, modify, and delete elements in a Go map.
Declaring and Initializing a Map
In Go, you can declare a map by specifying the key and value types within square brackets ([]
), followed by the map keyword. For example, let’s create a map to store the quantity of fruits:
var fruitQuantities map[string]int
Maps are reference types, so you need to initialize them before use. You can use the make function to create an empty map:
fruitQuantities = make(map[string]int)
Alternatively, you can declare and initialize a map in a single line:
fruitQuantities := make(map[string]int)
Inserting Elements into a Map
You can add key-value pairs to a map using the assignment operator (=
) or the map[key] = value
syntax:
fruitQuantities["apple"] = 5
fruitQuantities["banana"] = 3
fruitQuantities["cherry"] = 7
Retrieving Elements from a Map
To retrieve a value from a map, provide the corresponding key. If the key is present in the map, it returns the associated value; otherwise, it returns the zero value for the value type. For example:
appleQuantity := fruitQuantities["apple"] // Returns 5
bananaQuantity := fruitQuantities["banana"] // Returns 3
You can also check if a key exists in the map using a two-value assignment:
quantity, exists := fruitQuantities["orange"]
if exists {
fmt.Println("Quantity of oranges:", quantity)
} else {
fmt.Println("Orange not found in the map")
}
Modifying Values in a Map
Modifying the value associated with a key in a map is as simple as assigning a new value to that key:
fruitQuantities["apple"] = 10
Deleting Elements from a Map
To delete a key-value pair from a map, use the delete
function:
delete(fruitQuantities, "cherry")
Iterating Over a Map
You can iterate over the key-value pairs in a map using a for
loop with the range
keyword:
for fruit, quantity := range fruitQuantities {
fmt.Printf("%s: %d\n", fruit, quantity)
}
This loop iterates through each key-value pair in the fruitQuantities
map and prints them.
Conclusion
Maps are a versatile data structure in Go, allowing you to efficiently manage key-value pairs. They are commonly used in various programming tasks, such as counting occurrences, caching results, or creating data lookup tables. By understanding how to declare, initialize, insert, retrieve, modify, and delete elements in a map, you can harness the power of this data structure in your Go programs, making your code more efficient and readable.
package main
import "fmt"
func main() {
// Declare and create a map to store fruit quantities
fruitQuantities := make(map[string]int)
// Adding key-value pairs to the map
fruitQuantities["apple"] = 5
fruitQuantities["banana"] = 3
fruitQuantities["cherry"] = 7
// Accessing values by key
fmt.Println("Number of apples:", fruitQuantities["apple"])
fmt.Println("Number of bananas:", fruitQuantities["banana"])
// Modifying a value
fruitQuantities["apple"] = 10
// Deleting a key-value pair
delete(fruitQuantities, "cherry")
// Checking if a key exists in the map
_, exists := fruitQuantities["banana"]
fmt.Println("Is banana in the map?", exists)
// Iterating over the map
fmt.Println("Fruit quantities:")
for fruit, quantity := range fruitQuantities {
fmt.Printf("%s: %d\n", fruit, quantity)
}
}
Output:
Number of apples: 5
Number of bananas: 3
Is banana in the map? true
Fruit quantities:
apple: 10
banana: 3
This code covers all the concepts discussed in the article and provides a complete example of how to work with maps (hashmaps) in Golang, including declaration, initialization, insertion, retrieval, modification, deletion, and iteration.