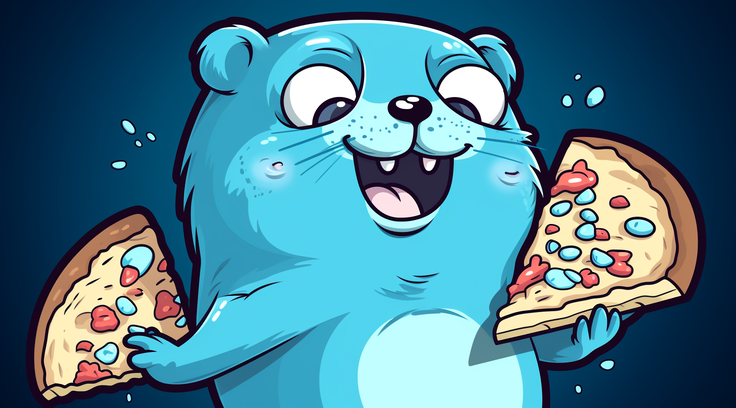
Simple Ways to Concatenate Slices in Golang
Introduction
Concatenating slices is a common and essential task in Go programming, helping you bring together data from multiple slices into a unified whole. In this article, we’ll walk through user-friendly methods to concatenate slices in Golang, making your code more versatile and efficient.
Understanding Slices in Golang
In Golang, a slice
is a dynamic array, a versatile data structure used for handling collections of elements. These slices are fundamental in Golang programming and play a crucial role in data manipulation.
For instance, imagine you have a list of numbers stored in a slice:
numbers := []int{1, 2, 3, 4, 5}
Slices provide flexibility, including features like appending, slicing, and concatenating. In this article, we’ll focus on concatenating slices in Golang, a valuable operation when you need to merge data from multiple slices. Whether you’re working with numbers, strings, or other data types, understanding how to combine slices efficiently will simplify your coding tasks. Let’s explore this process in detail.
Using the Append Function
The append
function is your trusty companion when it comes to merging slices in Go. It allows you to add one slice to another, resulting in a unified, combined slice.
package main
import "fmt"
func main() {
slice1 := []int{1, 2, 3}
slice2 := []int{4, 5, 6}
concatenatedSlice := append(slice1, slice2...)
fmt.Println(concatenatedSlice)
}
Output:
[1 2 3 4 5 6]
With the help of the spread operator (...
), you can concatenate slice1
and slice2
effortlessly. It’s a concise and clean way to combine slices in Golang.
Creating a Custom Concatenation Function
While the append
function is powerful, you may sometimes want more control over the concatenation process. Creating a custom function gives you that flexibility.
package main
import "fmt"
func concatenateStringSlices(slice1 []string, slice2 []string) []string {
result := append(slice1, slice2...)
return result
}
func main() {
slice1 := []string{"apple", "banana"}
slice2 := []string{"cherry", "date"}
concatenatedSlice := concatenateStringSlices(slice1, slice2)
fmt.Println(concatenatedSlice)
}
Output:
[apple banana cherry date]
In this example, we’ve created a custom function called concatenateStringSlices
specifically for []string
slices. It uses the append
function to merge two string slices, slice1
and slice2
. This approach gives you fine-grained control over concatenation and is particularly useful when dealing with slices of strings or other custom types.
By crafting custom functions like concatenateStringSlices
, you can concatenate slices of specific types and tailor the behavior to your needs. Flexibility at its finest!
Concatenation Considerations
As you venture further into concatenating slices, keep performance and memory allocation in mind. The append
function reallocates memory when needed, which can affect performance with large slices. Be mindful of these aspects when working with extensive data sets.
Conclusion
Concatenating slices in Golang is a fundamental skill for every developer. Whether you choose the built-in append
function or the spread operator, grasping these techniques will elevate your code. By picking the right method for your task, you can concatenate slices in Golang effortlessly, making your Go programming journey smoother and more productive. Happy coding!