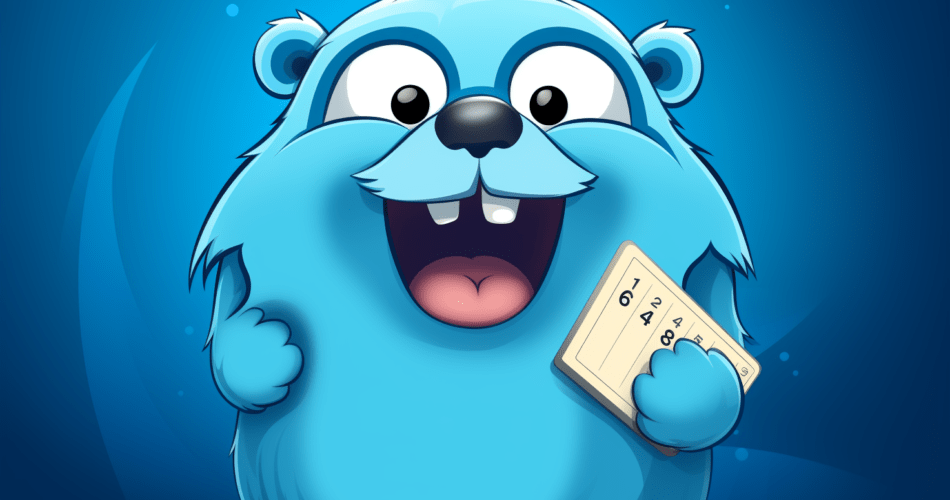
Efficient Error Handling in Golang
Introduction
Error handling in Golang is essential for creating reliable applications. This article explores best practices in Go error handling, focusing on efficiency and code stability. Whether you’re new to Go or a seasoned developer, you’ll gain valuable insights into handling errors effectively in your applications. Let’s dive into these practices to enhance your Go coding skills and create resilient software.
Error Handling Basics
Now that we’ve set the stage, let’s dive into the basics of error handling in Golang. Understanding these fundamentals is crucial for building robust Go applications while keeping your code clean and efficient.
In Go, errors are a part of life, and they come in two primary flavors: standard errors and custom errors.
1. Standard Errors:
These are pre-defined errors provided by the Go standard library. They offer basic information about what went wrong, making them easy to understand and handle. For example:
file, err := os.Open("example.txt")
if err != nil {
fmt.Println("Error:", err)
return
}
Here, if the file “example.txt” cannot be opened, the standard error value provides details about the issue.
2. Custom Errors:
Custom errors, on the other hand, are user-defined error types. You create them to provide more context and specific details about errors unique to your application. For instance:
type MyCustomError struct {
Message string
}
func (e MyCustomError) Error() string {
return e.Message
}
func main() {
err := MyCustomError{"Something went wrong"}
fmt.Println("Custom Error:", err)
}
In this example, we create a custom error type, MyCustomError, which includes a message to convey specific information about the error.
In Go, errors are represented as values rather than exceptions, meaning that when a function encounters an error, it returns an error value rather than throwing an exception. It’s then up to the calling code to check this value and decide how to handle the error.
Understanding these fundamental error types is key to effective error handling. In the next section, we’ll explore best practices to efficiently manage these errors and keep your Go code clean and concise.
Best Practices
Now that we’ve covered the basics of error handling in Golang, it’s time to delve into some best practices. These practices will help you manage errors efficiently and maintain clean and readable code in your Go applications.
1. Use Explicit Error Returns:
Go doesn’t rely on exceptions; instead, it encourages explicit error returns. Always return errors as values alongside your function’s main results. This clarity ensures that errors are an integral part of your function’s signature, making them impossible to ignore.
func divide(a, b float64) (float64, error) {
if b == 0 {
return 0, errors.New("division by zero")
}
return a / b, nil
}
2. Limit Error Checks in the Main Code Path:
Excessive error checks can clutter your code. Use control flow constructs like if statements to handle errors at the point where they matter most, keeping the main code path clean and focused.
result, err := someFunction()
if err != nil {
// Handle the error here
return
}
// Continue with the successful operation
3. Error Propagation and Context:
When passing errors up the call stack, wrap them with additional context to provide more information about the error’s origin. This practice helps in diagnosing issues quickly during debugging.
func readFile(filename string) ([]byte, error) {
data, err := ioutil.ReadFile(filename)
if err != nil {
return nil, fmt.Errorf("error reading file %s: %w", filename, err)
}
return data, nil
}
4. Logging and Error Reporting:
Know when to log errors and how to report them effectively. Logging is essential for debugging and monitoring your application in production. You can use libraries like log or external error reporting services to track and analyze errors.
5. Proper Use of Panic and Recover:
Use panic and recover judiciously. They are powerful tools but can lead to unmanageable code if misused. Reserve them for truly exceptional situations where normal error handling is not practical.
These best practices lay a solid foundation for handling errors efficiently in your Go applications. Embrace them to create code that not only functions correctly but is also easy to maintain and understand. In the next section, we’ll explore specific error handling patterns to further enhance your skills.
Error Handling Patterns
As we continue our journey into mastering error handling in Golang, it’s crucial to understand the various error handling patterns that can make your code both effective and maintainable. Let’s explore these patterns, each tailored to different scenarios.
1. Handling Errors in Control Structures:
if-else Statements: This is the most straightforward way to handle errors. Check for errors after calling a function and proceed accordingly. switch Statements: Use a switch statement to handle different error cases if you have multiple error conditions to evaluate.
result, err := someFunction()
if err != nil {
// Handle the error here
} else {
// Continue with the successful operation
}
2. Managing Multiple Errors:
Sometimes, you encounter multiple errors in a function. You can aggregate them using error variables and return them collectively. Alternatively, use libraries like multierror to manage multiple errors efficiently.
func doSomething() error {
var errs *multierror.Error
if err := operation1(); err != nil {
errs = multierror.Append(errs, err)
}
if err := operation2(); err != nil {
errs = multierror.Append(errs, err)
}
return errs.ErrorOrNil()
}
3. Error Handling in Goroutines:
When working with goroutines, it’s crucial to manage errors effectively. You can use channels to collect errors from concurrent operations and handle them appropriately.
These error handling patterns provide flexibility and clarity when dealing with errors in different contexts. By choosing the right pattern for your specific use case, you can ensure that your code remains robust and easy to maintain. In the next section, we’ll explore how to test error scenarios effectively.
func processFiles(files []string) error {
errChan := make(chan error, len(files))
for _, file := range files {
go func(filename string) {
if err := processFile(filename); err != nil {
errChan <- err
}
}(file)
}
for i := 0; i < len(files); i++ {
if err := <-errChan; err != nil {
// Handle the error
}
}
return nil
}
Conclusion
In conclusion, mastering error handling in Golang is essential for writing reliable Go code. By following best practices like explicit error returns, limiting checks, providing context, and using appropriate patterns, you’ll enhance code quality and maintainability. These skills are invaluable for any Go developer, so keep them in your toolkit as you venture into the world of Go programming. Happy coding!