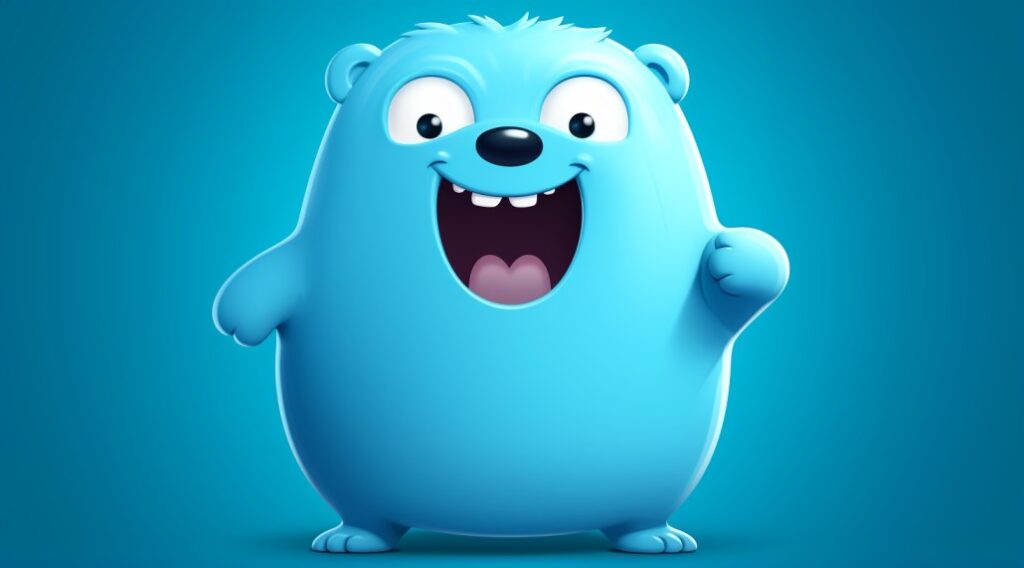
JSON in Golang: Best Practices and Examples
Table of Contents
- Introduction
- Understanding JSON in Golang
- Unmarshalling JSON Data
- Marshalling Data to JSON
- Working with Complex Data Structures (e.g., Maps, Slices)
- Handling JSON Maps
- Working with JSON Slices
- Conclusion
Introduction
JSON in Golang is a potent fusion for web development. In this comprehensive article, we’ll delve deep into harnessing the power of JSON data in the Go programming language. Drawing from my extensive experience as a developer, we’ll explore practical insights and examples to empower you in effectively working with JSON in Golang. Whether you’re a beginner or a seasoned coder, this guide will equip you with the skills and knowledge needed to master JSON in the Go programming language.
Understanding JSON in Golang
JSON in Golang involves efficiently handling JSON data using the Go programming language. JSON, or JavaScript Object Notation, is a universal data format for web communication due to its simplicity for humans and machines alike.
Golang simplifies JSON processing through its built-in encoding/json package, akin to a well-equipped toolbox. In essence, JSON in Golang revolves around harnessing this package’s capabilities for seamless JSON data interaction, including parsing, manipulation, and serialization.
In the following sections, we’ll lay the groundwork for effectively working with JSON in Golang, empowering you to decode, modify, and serialize JSON data effortlessly—a vital skillset for modern web development with Go. Let’s embark on this journey into the realm of JSON in Golang together.
Unmarshalling JSON Data
In the realm of JSON in Golang, unmarshalling JSON data is often the first step in the journey. Unmarshalling, or decoding, refers to the process of taking a JSON string and converting it into a structured format that Golang can understand. This structured format is typically a Go struct, which defines the data’s shape.
Take, for instance, this JSON snippet:
{
"name": "John Doe",
"age": 30,
"city": "New York"
}
With a corresponding Go struct like this:
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
City string `json:"city"`
}
And the encoding/json
package, we can seamlessly unmarshal the JSON data into our Go struct. Here’s how it’s done:
package main
import (
"encoding/json"
"fmt"
)
func main() {
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
City string `json:"city"`
}
jsonStr := `{
"name": "John Doe",
"age": 30,
"city": "New York"
}`
var person Person
if err := json.Unmarshal([]byte(jsonStr), &person); err != nil {
fmt.Println("Error:", err)
return
}
fmt.Println("Name:", person.Name)
fmt.Println("Age:", person.Age)
fmt.Println("City:", person.City)
}
Output :
Name: John Doe
Age: 30
City: New York
With this, we’ve successfully unmarshalled the JSON data into our Person struct, allowing us to work with it as native Go data.
Marshalling Data to JSON
In the realm of JSON in Golang, it’s not just about reading JSON; it’s also about creating it. Marshalling is the process of converting Go data structures, such as structs, into JSON format. This is invaluable when you want to send data as JSON in web applications or APIs.
Marshalling Go structs into JSON: To serialize data, you start with a Go struct that represents your data’s structure. Using the encoding/json package, you can marshal (encode) this Go struct into a JSON-formatted string. Here’s a simple example:
package main
import (
"encoding/json"
"fmt"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
City string `json:"city"`
}
func main() {
person := Person{
Name: "John Doe",
Age: 30,
City: "New York",
}
jsonData, err := json.Marshal(person)
if err != nil {
fmt.Println("Error:", err)
return
}
fmt.Println(string(jsonData))
}
Output:
{“name”:”John Doe”,”age”:30,”city”:”New York”}
This code snippet marshals our Person struct into a JSON-formatted string.
Customizing JSON output: Golang provides struct tags to customize the JSON output. By adding tags like json:“name” to struct fields, you can control the JSON key names. This flexibility is handy when your Go struct differs from the expected JSON structure.
Handling non-standard JSON data types: JSON can represent a variety of data types, including strings, numbers, booleans, arrays, and objects. Golang effortlessly handles these standard types, but sometimes you encounter non-standard or custom types. We’ll explore how to work with these scenarios, ensuring your Go application can marshal any data into JSON format.
Working with Complex Data Structures (e.g., Maps, Slices)
In the world of JSON in Golang, you’ll often encounter JSON data that goes beyond simple key-value pairs. It may include complex data structures like maps and slices. Golang equips you with powerful tools to navigate and work with these intricacies effectively.
Let’s delve into how you can tackle these complex data structures:
Handling JSON Maps
JSON often represents complex data using nested objects or maps. These can be deeply nested, creating a hierarchical structure. In Golang, you can efficiently work with such maps by defining corresponding Go structs.
Consider this JSON snippet:
{
"person": {
"name": "John Doe",
"age": 30
},
"address": {
"city": "New York",
"zip": 10001
}
}
To access the values within the “person” and “address” maps, you can define Go structs like this:
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
}
type Address struct {
City string `json:"city"`
Zip int `json:"zip"`
}
type Data struct {
Person Person `json:"person"`
Address Address `json:"address"`
}
Now, you can unmarshal the JSON data into the Data struct and access the values directly:
package main
import (
"encoding/json"
"fmt"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
}
type Address struct {
City string `json:"city"`
Zip int `json:"zip"`
}
type Data struct {
Person Person `json:"person"`
Address Address `json:"address"`
}
func main() {
var data Data
jsonData := []byte(`{
"person": {
"name": "John Doe",
"age": 30
},
"address": {
"city": "New York",
"zip": 10001
}
}`)
if err := json.Unmarshal(jsonData, &data); err != nil {
fmt.Println("Error:", err)
return
}
// Accessing values:
name := data.Person.Name
age := data.Person.Age
city := data.Address.City
zip := data.Address.Zip
fmt.Println("Name:", name)
fmt.Println("Age:", age)
fmt.Println("City:", city)
fmt.Println("Zip:", zip)
}
###Output:
Name: John Doe
Age: 30
City: New York
Zip: 10001
By defining appropriate Go structs and using them for unmarshalling, you make it more straightforward to work with complex JSON maps.
Working with JSON Slices
JSON arrays, represented as slices in Golang, can vary in length and content. Handling them dynamically is a core aspect of working with JSON in Golang.
Consider this JSON snippet:
{
"items": [1, 2, 3, 4, 5]
}
To work with the “items” slice in Golang, you can define a corresponding struct:
type Data struct {
Items []int `json:"items"`
}
Now, you can unmarshal the JSON data into the Data struct and access the slice directly:
package main
import (
"encoding/json"
"fmt"
)
type Data struct {
Items []int `json:"items"`
}
func main() {
var data Data
jsonData := []byte(`{
"items": [1, 2, 3, 4, 5]
}`)
if err := json.Unmarshal(jsonData, &data); err != nil {
fmt.Println("Error:", err)
return
}
// Accessing the slice:
items := data.Items
fmt.Println("Items:", items)
}
Output:
Items: [1 2 3 4 5]
By defining a struct that matches the JSON structure, you simplify the handling of JSON slices, regardless of their length or content.
Handling complex data structures in JSON is a valuable skill, and with Golang’s capabilities, you’re well-equipped to tackle these challenges efficiently. In the following sections, we’ll dive deeper into practical examples to solidify your understanding.
Conclusion
Mastering JSON in Golang is essential for seamless data handling in your projects. This guide has provided you with the core skills, from unmarshalling JSON data into native Go structures to marshalling Go data into JSON. You’ve also learned to work with complex data structures like maps and slices, ensuring readiness for real-world challenges.
Through practice and staying updated with evolving best practices, you’ll confidently tackle JSON-related tasks in your Golang projects. Harness the power of JSON to build robust web applications, APIs, and data-driven solutions within the expanding Golang ecosystem. Happy coding!