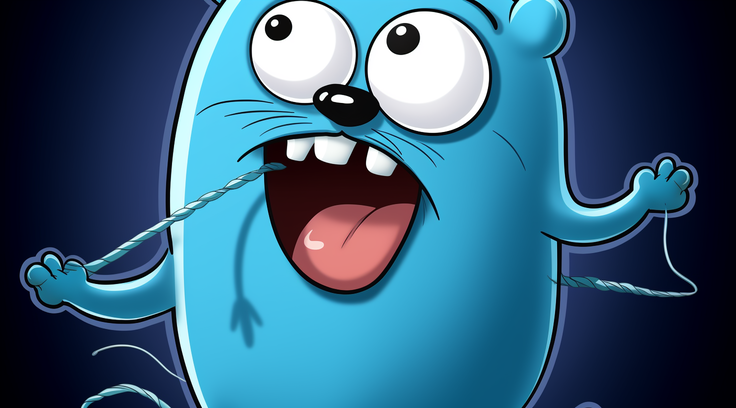
Mastering Go String Trimming
Introduction
In Go (Golang), strings play a pivotal role in text processing. Trimming strings, often referred to as Go string trimming, entails precision removal of undesirable leading and trailing whitespace or specified characters. This meticulous process greatly improves data quality, consistency, and visual appeal. This tutorial delves into Go string trimming techniques, exploring vital functions and methods. By mastering these skills, you’ll seamlessly enhance your code and achieve impeccable text manipulation proficiency in Go.
Table of Contents
- Introduction
- Method 1: Using strings.TrimSpace()
- Method 2: Custom Trimming with strings.Trim()
- Method 3: Trim Prefix and Suffix with strings.TrimPrefix() and strings.TrimSuffix()
- Conclusion
Method 1: Using strings.TrimSpace()
When it comes to streamlining your text manipulation tasks, the strings.TrimSpace()
function emerges as an indispensable asset. This versatile function excels in effortlessly removing extraneous whitespace characters from the beginning and end of a string, ensuring a cleaner and more uniform data representation.
package main
import (
"fmt"
"strings"
)
func main() {
text := " This is a string with spaces. "
trimmed := strings.TrimSpace(text)
fmt.Println("Original:", text)
fmt.Println("Trimmed:", trimmed)
}
Output :
Original: This is a string with spaces.
Trimmed: This is a string with spaces.
Method 2: Custom Trimming with strings.Trim()
While standard trimming methods work well, there are instances when a more tailored approach is required. Enter Go’s strings.Trim() function, designed to provide precise control over which characters to remove from a string. This dynamic function empowers you to shape your strings according to specific criteria, enhancing data accuracy and presentation.
package main
import (
"fmt"
"strings"
)
func main() {
text := "~~~Trim these tildes~~~"
trimmed := strings.Trim(text, "~")
fmt.Println("Original:", text)
fmt.Println("Trimmed:", trimmed)
}
Output :
Original: ~~~Trim these tildes~~~
Trimmed: Trim these tildes
Method 3: Trim Prefix and Suffix with strings.TrimPrefix() and strings.TrimSuffix()
When precision is paramount, Go’s strings.TrimPrefix() and strings.TrimSuffix() functions step in as reliable tools. These functions specialize in the meticulous removal of designated prefixes or suffixes from a string, ensuring that your data remains accurate and well-structured.
package main
import (
"fmt"
"strings"
)
func main() {
text := "Golang is amazing!"
withoutPrefix := strings.TrimPrefix(text, "Golang")
withoutSuffix := strings.TrimSuffix(text, "amazing!")
fmt.Println("Original:", text)
fmt.Println("Without Prefix:", withoutPrefix)
fmt.Println("Without Suffix:", withoutSuffix)
}
Output :
Original: Golang is amazing!
Without Prefix: is amazing!
Without Suffix: Golang is
Conclusion:
Trimming strings is an essential skill for effective text manipulation in Go. The strings.TrimSpace()
function simplifies whitespace removal, while strings.Trim()
, strings.TrimPrefix()
, and strings.TrimSuffix()
offer versatile customization. Whether for data cleaning, input validation, or formatting, string trimming enhances the reliability and functionality of your applications. Armed with this comprehensive guide, you’re ready to optimize your string handling in Go.