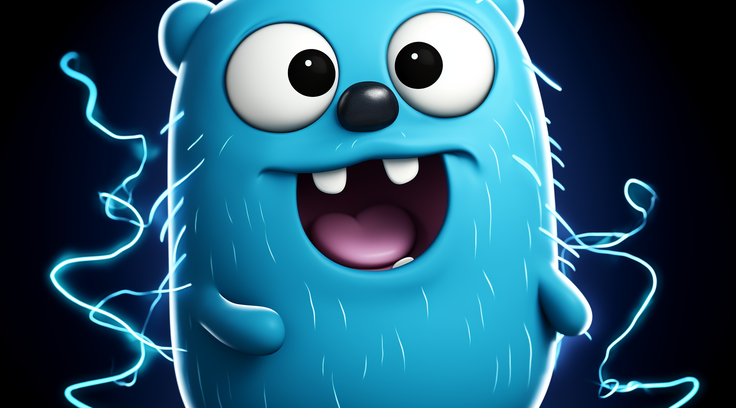
How to Creata a Multiline String in Golang
Multiline String in Golang is a fundamental concept used to store and manipulate text. Multiline strings are strings that span across multiple lines, often used for embedding blocks of text like documentation, SQL queries, or templates. While Go doesn’t have a built-in syntax for multiline strings like some other languages, there are several ways to achieve multiline strings effectively. In this tutorial, we’ll explore different methods to create multiline strings in Go.
Table of Contents:
- Using Raw String Literals
- Using String Concatenation
- Using Join Function
- Conclusion
Method 1: Using Raw String Literals
Raw string literals in Go allow you to include newline characters directly within the string without any escaping.
package main
import "fmt"
func main() {
multilineStr := `This is a multiline
string created using raw
string literals.`
fmt.Println(multilineStr)
}
Output:
This is a multiline
string created using raw
string literals.
Method 2: Using String Concatenation
You can create multiline strings by concatenating multiple string literals, each on a separate line.
package main
import "fmt"
func main() {
multilineStr := "This is a multiline\n" +
"string created using\n" +
"string concatenation."
fmt.Println(multilineStr)
}
Output:
This is a multiline
string created using
string concatenation.
Method 3: Using Join Function
The strings.Join()
function can be used to create a multiline string by joining an array of strings.
package main
import (
"fmt"
"strings"
)
func main() {
lines := []string{
"This is a multiline",
"string created using",
"the strings.Join() function.",
}
multilineStr := strings.Join(lines, "\n")
fmt.Println(multilineStr)
}
Output:
This is a multiline
string created using
the strings.Join() function.
Conclusion
In this tutorial, you’ve learned various methods to create multiline strings in Go. Each method offers a way to structure your text over multiple lines, catering to different coding styles and preferences. Choose the method that best suits your needs and helps maintain code readability. Happy coding!