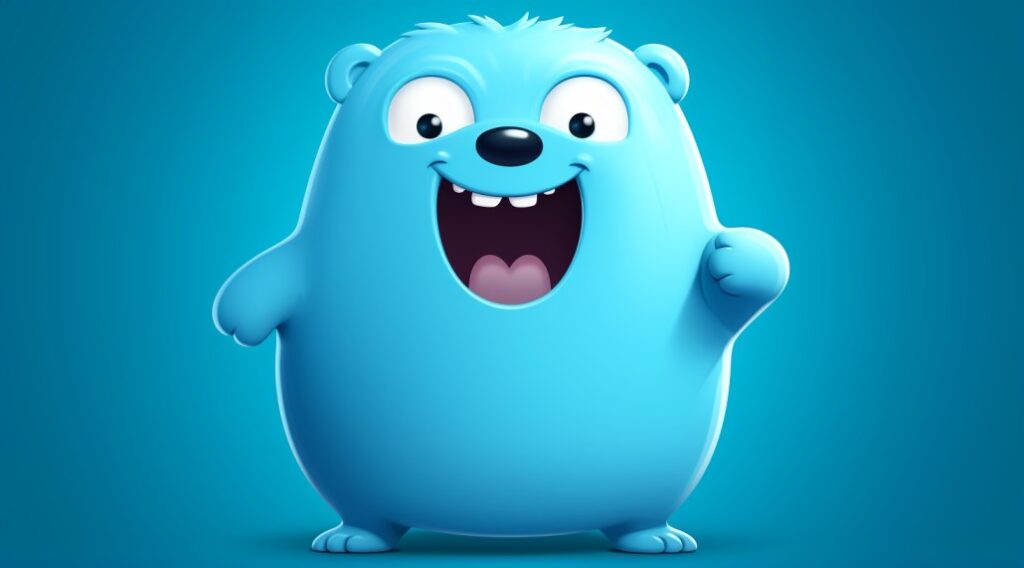
A Comprehensive Guide to Switch Statements in Golang
Introduction
Switch statements in Golang are a powerful tool that enable you to make decisions based on the value of an expression. These statements are incredibly flexible and offer a range of features to simplify your code and enhance its readability. In this comprehensive guide, we will delve into the world of switch statements in Golang and explore their numerous use cases, demonstrating how they can streamline your programming tasks and make your code more elegant and efficient.
1. Basic Switch Statement
The basic structure of a switch statement in Go looks like this:
switch expression {
case value1:
// Code to execute if expression equals value1
case value2:
// Code to execute if expression equals value2
default:
// Code to execute if expression doesn't match any case
}
2. Expressionless Switch
In Go, you can use a switch statement without an expression. This is useful for writing cleaner code when you need to evaluate multiple conditions. Example:
package main
import "fmt"
func main() {
age := 18
switch {
case age < 12:
fmt.Println("Child")
case age < 18:
fmt.Println("Teenager")
default:
fmt.Println("Adult")
}
}
Output:
Adult
3. Switch with Multiple Cases
You can include multiple values in a single case, separated by commas. Example:
package main
import "fmt"
func main() {
day := "Tuesday"
switch day {
case "Monday", "Tuesday", "Wednesday", "Thursday", "Friday":
fmt.Println("Weekday")
case "Saturday", "Sunday":
fmt.Println("Weekend")
}
}
Output:
Weekday
4. Fallthrough Statement
The fallthrough
statement transfers control to the next case without evaluating its condition. This can be useful in certain scenarios. Example:
package main
import "fmt"
func main() {
num := 1
switch num {
case 1:
fmt.Println("One")
fallthrough
case 2:
fmt.Println("Two")
case 3:
fmt.Println("Three")
}
}
Output:
One
Two
5. Type Switch
Type switches allow you to switch on the type of an interface value. This is particularly useful when working with interfaces. Example:
package main
import "fmt"
func main() {
var val interface{}
val = "Hello"
switch val.(type) {
case int:
fmt.Println("It's an integer")
case string:
fmt.Println("It's a string")
}
}
Output:
It’s a string
6. Switch with Initializer
You can use an initializer statement before the switch expression. This can help avoid repeating the same expression in each case. Example:
package main
import "fmt"
func main() {
switch num := 7; {
case num < 0:
fmt.Println("Negative")
case num > 0:
fmt.Println("Positive")
default:
fmt.Println("Zero")
}
}
Output:
Positive
7. Switch vs. if-else Chains
Switch statements and if-else chains are both control structures that allow you to make decisions based on certain conditions. They can be used interchangeably in many cases, but each has its strengths and weaknesses, and the choice between them depends on the context and the problem you’re trying to solve.
Switch Statements:
- Suitable for comparing a single expression against a fixed set of values.
- Ideal for cases where you need to determine the type of an expression using a type switch.
- Offers better readability when dealing with multiple cases.
- Can be more efficient than if-else chains in some scenarios due to potential compiler optimizations.
- Supports the fallthrough statement for controlled execution flow.
If-Else Chains:
- More flexible for handling complex conditions involving logical operators or multiple expressions.
- Better for conditions that involve ranges of values or require custom conditions.
- Offers greater control over the flow of execution.
- Can handle a wide variety of conditions, making it suitable for complex decision-making.
- Suitable when readability is important but the logic is not well-suited for switch cases.
When to Choose:
- Choose switch statements when dealing with a known set of values or when determining expression types.
- Choose if-else chains when conditions are complex, involve logical operators, or require custom conditions.
- Consider the nature of the problem, the specific requirements, and the desired readability when making your choice.
Ultimately, the decision between switch statements and if-else chains should be based on the complexity of conditions, the type of values being compared, and the readability and maintainability of your code. Both control structures have their strengths and are valuable tools in a programmer’s toolkit.
Conslusion
Switch statements are a versatile tool for making decisions in Go. They can handle various scenarios, from simple value matching to more complex type assertions. By mastering switch statements, you can write cleaner and more concise code that is easier to read and maintain. Happy Coding!