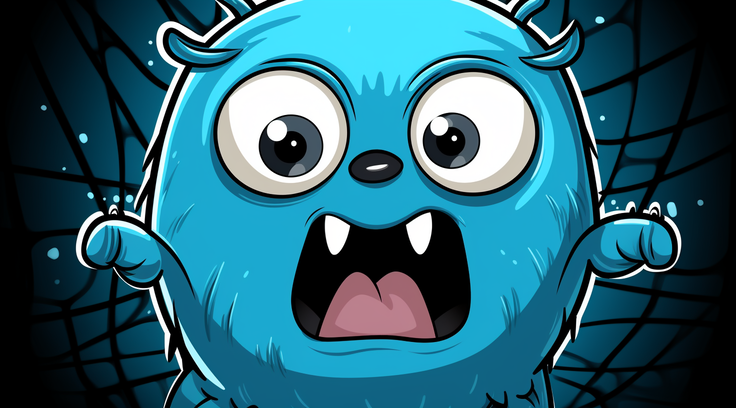
5 Ways to Split a String in Golang (With Examples)
In Go programming, efficiently dividing sentences using specific symbols can greatly enhance code functionality. Thankfully, Go equips developers with a versatile toolbox called ‘strings,’ which facilitates effective management of words and sentences. This comprehensive guide introduces five simple yet powerful methods for Split a String in Golang while optimizing your string manipulation tasks.
In this easy guide, we’ll learn five simple methods for splitting sentences in Go:
- Using the strings.Split() function
- Using the strings.Fields() function
- Using the strings.SplitN() function
- Using the strings.SplitAfter() function
- Using the strings.SplitAfterN()function
Method 1: Using the strings.Split() function
The strings.Split()
function is a cornerstone for splitting sentences in Go. Imagine having a list of fruits such as “apple,banana,orange,grape.” By using this function with a designated delimiter, the sentence transforms into a slice of substrings, as illustrated below:
package main
import (
"fmt"
"strings"
)
func main() {
input := "apple,banana,orange,grape"
delimiter := ","
parts := strings.Split(input, delimiter)
for _, part := range parts {
fmt.Println(part)
}
}
output
apple
banana
orange
grape
Method 2: Using the strings.Fields() function
When the need arises to split words using spaces and tabs while eliminating empty spaces, the strings.Fields()
function proves invaluable. Observe its functionality through the following example:
package main
import (
"fmt"
"strings"
)
func main() {
input := "This is a sample sentence with multiple words."
words := strings.Fields(input)
for _, word := range words {
fmt.Println(word)
}
}
output
This
is
a
sample
sentence
with
multiple
words.
Method 3: Using the strings.SplitN() function
For scenarios where controlled splitting is essential, the strings.SplitN()
function is your ally. Consider a string of colon-separated numbers like “one:two:three:four:five.” Here, the function can be harnessed to perform selective splitting:
package main
import (
"fmt"
"strings"
)
func main() {
input := "one:two:three:four:five"
delimiter := ":"
count := 3
parts := strings.SplitN(input, delimiter, count)
for _, part := range parts {
fmt.Println(part)
}
}
output
one
two
three:four:five
Method 4: Using the strings.SplitAfter() function
The strings.SplitAfter()
function excels at segmenting strings after specific substrings. Assume you have a text like “Hello!How are you?Goodbye!” and intend to split it post-question marks:
package main
import (
"fmt"
"strings"
)
func main() {
input := "Hello!How are you?Goodbye!"
delimiter := "?"
parts := strings.SplitAfter(input, delimiter)
for _, part := range parts {
fmt.Println(part)
}
}
output
Hello!How are you?
Goodbye!
Method 5: Using the strings.SplitAfterN() function
Similar to strings.SplitAfter()
, strings.SplitAfterN()
enables targeted string division after a defined count of occurrences. Imagine a series of dates like “2023-01-01_2023-02-01_2023-03-01.” By applying this function, you can achieve the following result:
package main
import (
"fmt"
"strings"
)
func main() {
input := "2023-01-01_2023-02-01_2023-03-01"
delimiter := "_"
count := 3
parts := strings.SplitAfterN(input, delimiter, count)
for _, part := range parts {
fmt.Println(part)
}
}
output
2023-01-01_
2023-02-01_
2023-03-01
To eliminate trailing underscores following a strings.SplitAfterN()
operation, a straightforward solution involves utilizing strings.TrimSuffix(part, delimiter)
. This technique efficiently removes the unwanted underscores from each resulting substring, as exemplified below:
package main
import (
"fmt"
"strings"
)
func main() {
input := "2023-01-01_2023-02-01_2023-03-01"
delimiter := "_"
count := 3
parts := strings.SplitAfterN(input, delimiter, count)
for i, part := range parts {
// Trim trailing underscores from each part
part = strings.TrimSuffix(part, delimiter)
parts[i] = part
fmt.Println(part)
}
}
output
2023-01-01
2023-02-01
2023-03-01
By using strings.TrimSuffix(part, delimiter)
, you remove the trailing underscore from each part before printing it. This way, you will get the split parts without the unwanted underscores.
Wrapping Up
In conclusion, this comprehensive guide has unveiled five efficient strategies to Split a String in Golang. These techniques, leveraging the robust functionalities of the ‘strings’ toolbox, are indispensable for managing words and sentences within Go programs. As you continue to explore and refine your skills in Go programming, these insights will undoubtedly prove invaluable. Happy coding, and may your coding journey be both productive and rewarding!