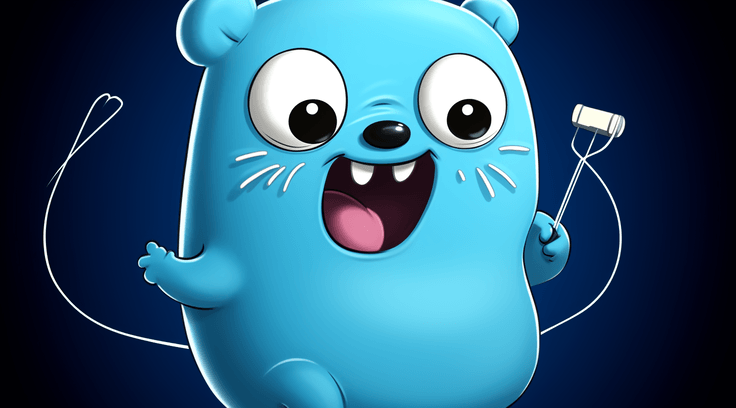
convert string into integer easily in golang
The Go programming language (Golang) has gained popularity due to its reputation for being easy to understand, facilitating concurrent and efficient processing. This characteristic has resulted in its extensive adoption among developers. An often encountered programming task is the conversion of a string into an integer. For instance, converting “5” into 5 can be highly advantageous, especially when dealing with user inputs or various types of information.
This guide aims to demonstrate the process of converting a string into an integer in Go.
Method 1: Using strconv.Atoi() Function
In Golang, you have access to a convenient built-in function called strconv.Atoi, which helps you convert strings into integers. This function takes a string as input and produces an integer as output. If the input string cannot be successfully converted into an integer, the function will generate an error.
Syntax :
func Atoi(s string) (int, error)
The return type of Atoi() function is (int, error), it returns the corresponding integer value, error (if any, else is nil).
Example 1 :
package main
import (
"fmt"
"strconv"
)
func main() {
// Convert string representations of base-10 integers to integers
// and print the results or errors
fmt.Println(strconv.Atoi("22"))
fmt.Println(strconv.Atoi("-22"))
fmt.Println(strconv.Atoi("67890"))
fmt.Println(strconv.Atoi("-67890"))
// Try to convert string representations of hexadecimal values to integers
// However, these conversions will fail due to non-numeric characters
fmt.Println(strconv.Atoi("abde"))
fmt.Println(strconv.Atoi("567ebc"))
}
Output 1 :
22 <nil>
-22 <nil>
67890 <nil>
-67890 <nil>
0 strconv.Atoi: parsing “abde”: invalid syntax
0 strconv.Atoi: parsing “567ebc”: invalid syntax
Example 2 :
package main
import (
"fmt"
"strconv"
)
func main() {
// Calling the convertAndPrint function with different input values
convertAndPrint("321") // Calling with base-10 integer value
convertAndPrint("-22") // Calling with negative base-10 integer value
convertAndPrint("2fb43") // Calling with hexadecimal string
}
// A function to convert a string to an int and print the result
func convertAndPrint(str string) {
// Using strconv.Atoi to convert the input string to an integer
fmt.Println("str:", str)
result, err := strconv.Atoi(str)
if err == nil {
fmt.Println("Converted Successfully, Result:", result)
} else {
fmt.Println("Failed to convert, Result:", result)
fmt.Println("Error:", err)
}
}
Output 2 :
str: 321
Converted Successfully, Result: 321
str: -22
Converted Successfully, Result: -22
str: 2fb43
Failed to convert, Result: 0
Error: strconv.Atoi: parsing “2fb43”: invalid syntax
Method 2: Using strconv.ParseInt() Function Golang also provides another function, strconv.ParseInt, which serves the same purpose. We can use this function to convert a string into an integer type.
Syntax :
func ParseInt(str string, base int, bitSize int)
In this context, the ‘str’ represents the string that you wish to convert. The ‘base’ parameter indicates the numeric base (ranging from 2 to 36) in which the string’s enclosed number is portrayed. For example, a base of 0 decodes the string’s prefix as 0x for hexadecimal, 0 for octal, and 0b for binary representations. When no prefix is present, the function assumes a base of 10. Finally, the ‘bitSize’ parameter determines the bit size of the resulting integer (options available: 0, 8, 16, 32, or 64).
Example :
package main
import (
"fmt"
"strconv"
)
func main() {
str := "768"
if i, err := strconv.ParseInt(str, 10, 32); err == nil {
fmt.Println("Converted Integer:", i)
} else {
fmt.Println("Error:", err)
}
}
Output :
Converted Integer: 768
The code above demonstrates the utilization of the string “768” to exemplify the conversion into an integer using the strconv.ParseInt function. This conversion occurs by explicitly specifying a base of 10 and a bit size of 32. Subsequently, the resulting integer is showcased on the console. If any problems emerge during the conversion process, the code will promptly generate an error message.
Conclusion
In conclusion, Golang’s strconv package equips developers with effective tools for converting strings into integers. Whether using strconv.Atoi for straightforward conversions or strconv.ParseInt for more complex scenarios involving different bases and bit sizes, these functions enhance the language’s flexibility. As a result, developers can adeptly handle user inputs, file operations, and data manipulations, elevating their coding proficiency within the Go programming realm.